Learn Python: How To Check If a List Is Empty?
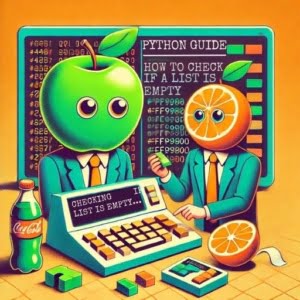
Checking if a list is empty in Python is sometimes needed when we validate data sets for scripts at IOFLOOD. In our experience, this task ensures that data processes can proceed accurately by confirming the presence of list elements. Today’s article explains how to check if a list is empty in Python, providing valuable insights and examples to assist our dedicated server customers.
In this guide, we’ll demystify the process of checking if a list is empty in Python. We’ll start from the basics and gradually delve into more advanced techniques. So, whether you’re a beginner just starting out or an intermediate user looking to brush up your skills, this guide has got you covered.
Let’s dive in and learn about empty list evaluations!
TL;DR: How Do I Check If a List is Empty in Python?
You can check if a list is empty in Python by using the
not
operator with the syntax,if not my_list:
. This operator returnsTrue
if the list is empty andFalse
otherwise.
Here’s a simple example:
my_list = []
if not my_list:
print('List is empty')
# Output:
# 'List is empty'
In this example, my_list
is an empty list. The not
operator checks if my_list
is empty, and if it is, it prints ‘List is empty’.
This is a quick and easy way to check if a list is empty in Python, but there’s more to learn! Stay tuned for more detailed explanations and advanced usage scenarios.
Table of Contents
Basic List Checks with ‘not’ Operator
In Python, the not
operator is one of the simplest and most common ways to check if a list is empty. The not
operator returns True
if the list is empty and False
if it’s not.
Let’s look at an example:
product_list = ["Apple", "Banana", "Cherry"]
product_list.clear() # This will empty the list
if not product_list:
print("The product list is now empty.")
# Output:
# 'The product list is now empty.'
In this code, we have a product_list
that initially contains some items. We clear the list using the clear()
function which removes all items from the list. After that, we use the not
operator to check if product_list
is now empty. If it is, we print ‘The product list is now empty.’
Advantages of the ‘not’ Operator
The not
operator is straightforward and easy to use, making it ideal for beginners. It’s also efficient, as it doesn’t need to iterate over the list to check if it’s empty.
If the variable is
None
or a non-list type, thenot
operator will still returnTrue
when used in this way. It’s always a good practice to ensure the variable is a list before performing a list-specific operation.
Checking Complex Data Structures
As you start dealing with more complex data structures like nested lists, the simple not
operator might not suffice. Similarly, when a list variable could potentially be None
, additional checks are necessary to avoid errors. Let’s explore these scenarios.
Checking Nested Lists
Consider a nested list that contains other lists as its elements. A simple not
check will only verify if the outer list is empty, not the inner lists. Here’s an example:
nested_list = [[]]
if not nested_list[0]:
print('Inner list is empty')
# Output:
# 'Inner list is empty'
In this code, nested_list
is a list containing one empty list. The not
operator checks if the first list inside nested_list
is empty, and if it is, it prints ‘Inner list is empty’.
Dealing with None Values
If your list variable might be None
, it’s important to check this before checking if it’s empty to avoid a TypeError
. Here’s how you can do it:
my_list = None
if my_list is None or not my_list:
print('Variable is None or list is empty')
# Output:
# 'Variable is None or list is empty'
In this example, my_list
is None
. The if
statement first checks if my_list
is None
, and if it’s not, it checks if my_list
is empty. If either condition is True
, it prints ‘Variable is None or list is empty’.
Alternative Methods to Check Lists
While using the not
operator is a common approach, Python offers other ways to check if a list is empty. These methods can be more suitable in certain scenarios or according to personal coding style.
Let’s explore two of these alternatives: using the len()
function and comparing the list to an empty list.
Using the len()
Function
The len()
function returns the number of items in a list. If the list is empty, len()
returns 0. Here’s an example:
my_list = []
if len(my_list) == 0:
print('The list is empty')
# Output:
# 'The list is empty'
In this code, my_list
is an empty list. The len()
function checks the length of my_list
, and if it’s 0, it prints ‘The list is empty’. This method is explicit and easy to understand, but it’s slightly less efficient than the not
operator as it counts the items in the list.
Comparing the List to an Empty List
Another method is to directly compare the list to an empty list ([]
). If they’re equal, the list is empty. Here’s how you can do it:
my_list = []
if my_list == []:
print('The list is empty')
# Output:
# 'The list is empty'
In this example, my_list
is an empty list. The if
statement checks if my_list
is equal to an empty list, and if it is, it prints ‘The list is empty’. This method is also explicit and easy to understand, but like the len()
method, it’s slightly less efficient than the not
operator.
Each of these methods has its advantages and disadvantages, and the best one to use depends on your specific scenario and personal preference. The
not
operator is generally the most efficient, but thelen()
function and list comparison methods can be more explicit and easier to understand for beginners.
Handling Errors in List Evaluation
While checking if a list is empty in Python is generally straightforward, you may encounter some common issues, especially when dealing with None
values or mutable default arguments. Let’s discuss these scenarios and provide some practical solutions.
Dealing with None Values
As we’ve seen earlier, if your list variable could potentially be None
, it’s important to check this before checking if it’s empty to avoid a TypeError
. Here’s a reminder of how you can do it:
my_list = None
if my_list is None or not my_list:
print('Variable is None or list is empty')
# Output:
# 'Variable is None or list is empty'
In this code, my_list
is None
. The if
statement first checks if my_list
is None
, and if it’s not, it checks if my_list
is empty. If either condition is True
, it prints ‘Variable is None or list is empty’.
Handling Mutable Default Arguments
In Python, if you’re using a mutable object like a list as a default argument in a function, it can lead to unexpected behavior. This is because the default argument is only evaluated once, not every time the function is called.
For instance, consider a function that appends an item to a list. If the list is a default argument and you don’t provide a list when calling the function, it will append the item to the same list every time, not a new list.
Here’s an example:
def append_to_list(item, my_list=[]):
my_list.append(item)
return my_list
print(append_to_list('apple')) # Output: ['apple']
print(append_to_list('banana')) # Output: ['apple', 'banana']
To avoid this, you can use None
as the default value and create a new list in the function if None
is provided:
def append_to_list(item, my_list=None):
if my_list is None:
my_list = []
my_list.append(item)
return my_list
print(append_to_list('apple')) # Output: ['apple']
print(append_to_list('banana')) # Output: ['banana']
These are some of the common issues you might encounter when checking if a list is empty in Python. Always be mindful of these potential pitfalls and use the appropriate checks and techniques to ensure your code behaves as expected.
Understanding Boolean Evaluation
Before we delve deeper into checking if a list is empty in Python, let’s take a step back and understand the fundamentals of Python’s list data type and how Python evaluates empty sequences.
Python Lists
In Python, a list is a data type that stores an ordered collection of items, which can be of any type. Here’s an example of a list:
my_list = ['apple', 'banana', 'cherry']
print(my_list)
# Output:
# ['apple', 'banana', 'cherry']
In this example, my_list
is a list of strings. Lists are defined by enclosing a comma-separated sequence of items in square brackets ([]
).
Boolean Evaluation of Empty Sequences
In Python, empty sequences are considered False
in a boolean context, while non-empty sequences are considered True
. This is why we can use the not
operator to check if a list is empty. When we use not
with a list, Python checks if the list is empty. If it is, not
returns True
; if the list is not empty, not
returns False
.
Here’s an example:
empty_list = []
non_empty_list = ['apple']
print(bool(empty_list)) # Output: False
print(bool(non_empty_list)) # Output: True
In this code, empty_list
is an empty list, and non_empty_list
is a list with one item. The bool()
function returns False
for empty_list
and True
for non_empty_list
.
Understanding these core concepts is crucial to grasp why the techniques for checking if a list is empty in Python work the way they do.
Further Resources for Python
Checking if a list is empty is just one of the many things you can do with lists in Python. There are other powerful concepts like list comprehension and various list methods that you can explore to leverage the full potential of lists in Python. If you’re interested in these concepts and more ways to utilize the Python language, here are a few resources that you might find helpful:
- Python Lists Tutorial: A comprehensive guide that covers Python lists from basics to advanced techniques.
Flattening a Nested List in Python: A tutorial demonstrating various methods to flatten nested lists in Python.
Using Empty Lists in Python: A guide that provides an overview of using empty lists in Python, including creating, checking, and appending elements to empty lists.
Python Official Documentation: Data Structures: The official Python documentation provides an in-depth tutorial on data structures, including lists, tuples, sets, and dictionaries.
W3Schools Python Lists Tutorial: This tutorial on W3Schools offers a beginner-friendly introduction to Python lists.
Python – Check if a List is Empty or Not: An article on GeeksforGeeks that demonstrates different approaches to check if a list is empty or not in Python.
Check if a List is Empty in Python: A Python programming example on Programiz that illustrates techniques to check if a list is empty in Python.
Recap: Evaluating Empty Python Lists
Throughout this guide, we’ve explored various methods to check if a list is empty in Python. We started with the simple yet powerful not
operator, which returns True
when a list is empty and False
otherwise.
We also discussed potential issues with this method, such as when dealing with None
values or nested lists, and provided solutions for these scenarios.
Then, we delved into alternative methods, like using the len()
function and comparing the list to an empty list. While these methods are slightly less efficient, they can be more explicit and easier to understand for beginners.
Finally, we discussed some common issues you might encounter when checking if a list is empty, such as dealing with mutable default arguments, and provided practical solutions.
In summary, checking if a list is empty in Python is a fundamental skill that plays a crucial role in various real-world scenarios. Whether you’re a beginner just starting out or an experienced developer looking to brush up your skills, understanding these methods can greatly enhance your Python coding prowess.