Python Switch Case | Using Switch Statements in Python
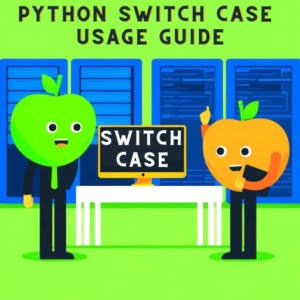
Proper usage of the switch-case structure in Python programming is imperative while managing systems at IOFLOOD, facilitating efficient conditional branching and code organization. In our experience, switch-case structures offer a concise and readable way to handle multiple conditions, enhancing code clarity and maintainability. Today’s article explores what a switch-case structure is in Python, providing examples and explanations to empower our customers with valuable scripting techniques for their cloud server hostings.
In this blog post, we’ll dive deep into these alternatives, explaining how you can implement switch case
structures in Python using various methods.
So, fasten your seatbelts, and let’s embark on this coding journey!
TL;DR What is a Switch Case Structure?
In programming, a
switch case
structure is a type of control statement that allows a variable to be tested for equality against a list of values. Each of these values is referred to as acase
, and the variable that is beingswitched on
is checked for eachcase
.
It’s akin to a more efficient version of an if-elif-else
structure. Rather than evaluating each condition sequentially until a true condition is found, a switch case
leaps directly to the branch corresponding to the ‘switched-on’ value. It’s like a multi-destination train where each stop represents a case, and the train (program) directly goes to the stop (case) that matches the passenger’s ticket (input value).
Table of Contents
Python Switch Case Statement Alternatives
Now, here’s the twist: Python, unlike many other programming languages, doesn’t have a built-in switch case
structure. You might wonder, ‘Why not?’ The philosophy of Python revolves around simplicity and readability, and the creators of Python believed that if-elif-else
statements were more aligned with this philosophy.
While if-elif-else
statements can certainly serve the purpose, there are scenarios where a switch case
structure can be a more efficient and elegant solution. This is especially true when dealing with a large number of conditions. In such cases, if-elif-else
statements can become unwieldy and hard to maintain.
This is where the flexibility of Python comes into play. Python offers several alternatives to implement the functionality of a switch case
structure.
Python’s ‘Match Case’: A Game Changer
With Python 3.10, a new feature was introduced that could potentially revolutionize how we handle condition checking in Python: the match case
statement. This new construct bears some resemblance to a switch case
structure, but it’s far more powerful and flexible.
How ‘Match Case’ Exceeds Traditional Switch Cases
The match case
statement in Python is not just a simple switch case
structure. It’s a comprehensive pattern matching mechanism that can match patterns of different shapes, destructure data, and even check for specific properties. This makes it a more versatile and potent tool than traditional switch case
structures.
A Practical Example of Using ‘Match Case’
Here’s another example of using match case
in Python:
def process_data(data):
match data:
case ('info', message):
print(f'Info: {message}')
case _:
print('Unknown data')
process_data(('info', 'All is well')) # Output: Info: All is well
process_data(('error', 'Something went wrong')) # Output: Unknown data
To better understand how the match case
statement works in Python, let’s consider the following example:
def process_data(data):
match data:
case ('error', message):
print(f'Error: {message}')
case ('warning', message):
print(f'Warning: {message}')
case _:
print('Unknown data')
process_data(('error', 'Something went wrong')) # Output: Error: Something went wrong
process_data(('info', 'All is well')) # Output: Unknown data
In this example, the process_data
function receives a tuple data
and uses a match case
statement to check the first element of the tuple. If it’s 'error'
or 'warning'
, it prints an appropriate message. If it’s anything else, it prints 'Unknown data'
. This shows how match case
can be used to handle various scenarios in a straightforward and efficient manner.
The Power and Simplicity of ‘Match Case’
The match case
statement in Python is a powerful tool that marries the efficiency of a switch case
structure with the versatility of pattern matching. It’s also easy to use and understand, making it an excellent addition to any Python programmer’s toolkit.
‘Match Case’: An Effective Alternative to Traditional Switch Cases
In conclusion, Python’s match case
statement is a powerful and user-friendly alternative to traditional switch case
structures. It’s a testament to Python’s flexibility and innovation, and it’s another reason why Python is such a fantastic language for both novices and seasoned programmers alike.
Using If/Elif Statements as an Alternative
As mentioned before, the Python “native” alternative to a switch case statement is if/elif.
Here’s an example of using if-elif-else
statements as a switch case
alternative:
def switch_case_if(value):
if value == 'case1':
return 'This is case 1'
elif value == 'case2':
return 'This is case 2'
elif value == 'case3':
return 'This is case 3'
else:
return 'This is the default case'
print(switch_case_if('case1')) # Output: This is case 1
print(switch_case_if('case4')) # Output: This is the default case
The most straightforward alternative to a switch case
is to use if-elif-else
statements. This is a simple and readable solution, but as mentioned before, it can become cumbersome when dealing with a large number of conditions.
Dictionaries as Switch Case Structures
Python’s dictionaries are versatile and potent data structures. They map keys to values, and this feature can be harnessed to implement a switch case structure. Instead of cases
, we have keys, and instead of switching
, we retrieve the value associated with a specific key.
Let’s illustrate this with an example:
def switch_case_dict(value):
return {
'case1': 'This is case 1',
'case2': 'This is case 2',
'case3': 'This is case 3'
}.get(value, 'This is the default case')
print(switch_case_dict('case1')) # Output: This is case 1
print(switch_case_dict('case4')) # Output: This is the default case
In this example, we define a function switch_case_dict
that accepts a value
as an argument. Inside the function, we create a dictionary where each key-value pair represents a case. The get()
method retrieves the value associated with the given value
. If the value
isn’t found in the dictionary, the get()
method returns a default value, which in this case is 'This is the default case'
.
Advantages of Dictionaries in Switch Case Structures
Using dictionaries as switch case structures comes with several benefits. First, it’s a neat and elegant solution that is easy to read and understand. Second, it’s highly efficient, as retrieving a value from a dictionary is a constant time operation, regardless of the dictionary’s size. This can lead to significant performance improvements when dealing with a large number of cases.
Using Getattr for Switch Case Structures
Another robust way to implement a switch case structure in Python is by using the getattr
function within classes. The getattr
function allows you to retrieve the value of a named attribute of an object. By defining methods in a class that correspond to different cases, you can use getattr
to call the appropriate method based on the ‘switched-on’ value.
Here’s an illustrative example:
class SwitchCaseClass:
def case1(self):
return 'This is case 1'
def case2(self):
return 'This is case 2'
def case3(self):
return 'This is case 3'
def default(self):
return 'This is the default case'
def switch_case_getattr(value):
switcher = SwitchCaseClass()
return getattr(switcher, value, switcher.default)()
print(switch_case_getattr('case1')) # Output: This is case 1
print(switch_case_getattr('case4')) # Output: This is the default case
In this example, we define a class SwitchCaseClass
with methods that correspond to different cases. We then define a function switch_case_getattr
that uses getattr
to call the appropriate method of SwitchCaseClass
based on the value
given. If the value
does not correspond to any method, getattr
calls a default method.
Further Resources for Python Iterator Mastery
As you continue your exploration of Python’s control structure, the following resources may prove immensely helpful:
- Python Loop Types Demystified – Learn about nested loops and their role in handling complex tasks.
Python do-while – Discover the key differences between do-while and other loop types in Python
Using “pass” in Python – Explore how “pass” helps structure code and create empty functions or classes.
Python ‘While’ Loop Guide – A guide by Real Python that provides an in-depth exploration of Python’s ‘while’ loop.
Iterators and Generators in Python – A Plain English guide that dives into the concept of iterators and generators in Python.
Generators in Python Simplified – An article on Medium breaking down the concept and usage of generators in Python.
Take advantage of these insights and make strides in mastering the intricate art of Python control structures and statements.
Wrapping Up: The Power of Python’s Flexibility
Throughout this post, we’ve embarked on a comprehensive journey exploring switch case structures in Python. While Python may not come with a built-in switch case
structure like some other languages, it certainly does not leave us in the lurch. Python offers a plethora of powerful alternatives that can help us achieve the same functionality, and often with added benefits.
We delved into how we can use if-elif-else
statements as a straightforward alternative to switch case
structures. We also discovered how to leverage Python’s dictionaries and the getattr
function to implement efficient and elegant switch case structures. These methods not only enhance the readability and maintainability of our code, but they can also improve its performance.
Furthermore, we got a glimpse of Python’s match case
statement, a powerful new feature introduced in Python 3.10. This versatile tool takes condition checking in Python to a whole new level, offering a robust pattern matching mechanism that outperforms traditional switch case
structures.
Need a refresher? See here.
In conclusion, Python’s flexibility and innovation shine through in its approach to switch case structures. Whether you’re a beginner or an experienced programmer, understanding these alternatives can significantly enhance your coding skills and open up new possibilities for writing efficient, readable, and maintainable Python code.