How to Sort a List in Java | Useful Methods and Examples
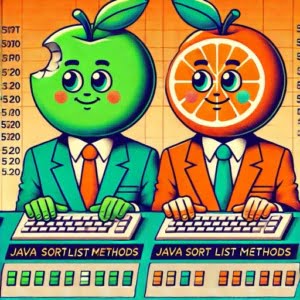
Sorting lists in Java is a fundamental operation we often perform at IOFLOOD to organize data and improve processing efficiency. Various Java list sort methods provide flexible and powerful ways to sort lists according to different criteria. Today’s article will explore how to sort a list in Java, offering practical examples and best practices to assist our dedicated server customers in managing their data effectively.
In this guide, we’ll walk you through the Java sort list processes, from the basics to more advanced techniques. We’ll cover everything from using the Collections.sort()
method, handling sorting with custom objects, to discussing alternative approaches.
Let’s dive in and start mastering list sorting in Java!
TL;DR: How Do I Sort a List in Java?
The simplest way to sort a list in Java is by using the
Collections.sort()
method. This method sorts the specified list into ascending order, according to the natural ordering of its elements.
Here’s a simple example:
List<Integer> numbers = Arrays.asList(3, 2, 1);
Collections.sort(numbers);
System.out.println(numbers);
// Output:
// [1, 2, 3]
In this example, we have a list of integers that we want to sort in ascending order. We use the Collections.sort()
method to sort the list, and then print the sorted list to the console. The output shows the list sorted in ascending order.
This is a basic way to sort a list in Java, but there’s much more to learn about sorting lists, especially when dealing with custom objects or when you want to sort in a different order. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
The Basics: How to Sort a List in Java
When dealing with lists in Java, one of the most basic and commonly used methods to sort a list is Collections.sort()
. This method sorts the elements of the list in ascending order according to their natural ordering.
Here’s a simple example of how it works:
List<String> fruits = Arrays.asList('Orange', 'Apple', 'Banana');
Collections.sort(fruits);
System.out.println(fruits);
// Output:
// [Apple, Banana, Orange]
In this example, we have a list of fruits that we want to sort in alphabetical order. We use the Collections.sort()
method to sort the list, we then print to the console the new sorted list. The output shows the list sorted in alphabetical order.
The Collections.sort()
method works well with lists of objects that implement the Comparable
interface, like String, Integer, and Date. It’s a simple, quick way to sort a list in Java.
However, it’s not without its limitations. The Collections.sort()
method sorts in ascending order by default, and it can’t handle null values. If you try to sort a list with null values, it will throw a NullPointerException
. Moreover, it may not work as expected with custom objects, unless the custom class implements the Comparable
interface and overrides the compareTo()
method.
Java Sort Custom Objects in Lists
When dealing with custom objects in Java, sorting a list can be a bit more complex. Thankfully, Java provides the Comparator
interface, which gives us more control over how our lists are sorted.
With a Comparator
, we can define custom sorting rules for our lists. Let’s take a look at an example where we have a list of Person
objects that we want to sort by name:
class Person {
String name;
// constructor, getters and setters omitted for brevity
}
List<Person> people = Arrays.asList(
new Person('Charlie'),
new Person('Alice'),
new Person('Bob')
);
Collections.sort(people, new Comparator<Person>() {
@Override
public int compare(Person p1, Person p2) {
return p1.getName().compareTo(p2.getName());
}
});
for (Person p : people) {
System.out.println(p.getName());
}
// Output:
// Alice
// Bob
// Charlie
In this example, we’ve created a custom Comparator
that compares Person
objects based on their names. We then pass this Comparator
to the Collections.sort()
method to sort our list of people. The output shows the names of the people in alphabetical order.
This approach is powerful because it lets you define custom sorting rules, but it can also be more complex and verbose. It also doesn’t handle null values by default, so you’ll need to add extra checks if your list might contain nulls.
To learn more about using Java Interfaces, you can refer to the article we posted on the subject, Here.
Alternate Java List Sort Methods
While Collections.sort()
and Comparator
are versatile and powerful, Java offers other methods and classes for sorting lists. Let’s explore some of these alternatives.
Using Arrays.sort()
The Arrays.sort()
method is another way to sort arrays in Java. It works similarly to Collections.sort()
, but it’s used for arrays instead of lists.
int[] numbers = {3, 2, 1};
Arrays.sort(numbers);
System.out.println(Arrays.toString(numbers));
// Output:
// [1, 2, 3]
In this example, we use Arrays.sort()
to sort an array of integers. The output shows the array sorted in ascending order.
How to Sort a List in Java With Stream.sorted()
Features in Java 8 included the Stream API, which provides a sorted()
method that returns a stream consisting of the elements of the original stream, sorted according to natural order.
List<String> fruits = Arrays.asList('Orange', 'Apple', 'Banana');
List<String> sortedFruits = fruits.stream()
.sorted()
.collect(Collectors.toList());
System.out.println(sortedFruits);
// Output:
// [Apple, Banana, Orange]
In this example, we create a stream from the list, sort it using the sorted()
method, and collect the result back into a list. The output shows the list sorted in alphabetical order.
Java Sort List With Third-Party Libraries
There are also third-party libraries like Google’s Guava that provide additional sorting capabilities. For example, Guava’s Ordering
class provides a fluent API for building complex comparators.
List<String> fruits = Arrays.asList('Orange', 'Apple', 'Banana');
List<String> sortedFruits = Ordering.natural().sortedCopy(fruits);
System.out.println(sortedFruits);
// Output:
// [Apple, Banana, Orange]
In this example, we use Guava’s Ordering
class to sort a list of strings. The output shows the list sorted in alphabetical order.
Each of these methods has its own benefits and drawbacks. Arrays.sort()
is great for arrays, Stream.sorted()
provides a functional programming approach, and Guava’s Ordering
offers a powerful, fluent API. However, they all require understanding and careful use to avoid pitfalls like null pointer exceptions or unexpected behavior with custom objects.
Solving Issues In Java List Sorting
Like any programming task, sorting lists in Java can encounter some common issues. Let’s discuss these problems and their solutions.
Handling Null Values
As mentioned earlier, Collections.sort()
and Comparator
do not handle null values by default. Trying to sort a list with null values will result in a NullPointerException
.
List<String> fruits = Arrays.asList('Orange', null, 'Banana');
Collections.sort(fruits);
// Output:
// NullPointerException
One solution is to use Java 8’s Comparator interface methods Comparator.nullsFirst()
or Comparator.nullsLast()
methods, which handle null values gracefully:
List<String> fruits = Arrays.asList('Orange', null, 'Banana');
Collections.sort(fruits, Comparator.nullsFirst(String::compareTo));
System.out.println(fruits);
// Output:
// [null, Banana, Orange]
Sorting Custom Objects Without Implementing Comparable
If you try to sort a list of custom objects without implementing the Comparable
interface, Collections.sort()
will throw a ClassCastException
:
class Person {
String name;
// constructor, getters and setters omitted for brevity
}
List<Person> people = Arrays.asList(
new Person('Charlie'),
new Person('Alice'),
new Person('Bob')
);
Collections.sort(people);
// Output:
// ClassCastException
The solution is to implement the Comparable
interface and override the compareTo()
method in your custom class, or to provide a custom Comparator
when calling Collections.sort()
.
Avoiding Performance Pitfalls
While sorting is a common operation, it can be a performance bottleneck if not done correctly. For large lists, consider using parallel sorting with Arrays.parallelSort()
or parallel streams. Also, when sorting complex objects, consider the cost of comparison operations and try to minimize them for better performance.
Understanding Java Sort Algorithms
Sorting is a fundamental concept in computer science and a critical operation in many applications. From organizing data in databases to ordering search results on a website, sorting algorithms play a crucial role in the efficient functioning of numerous systems.
At its core, a sorting algorithm orders the elements of a list based on a certain attribute. This could be numerical value, alphabetical order, or any other property that has a defined order. The efficiency of a sorting algorithm is typically measured in terms of its time complexity, which is a measure of the amount of time it takes to sort a list as a function of the list’s length.
Java provides several built-in methods for sorting lists, each utilizing different sorting algorithms. For example, the Collections.sort()
method uses a variant of the MergeSort algorithm, which is efficient but can be overkill for small lists. On the other hand, the Arrays.sort()
method uses a variant of the QuickSort algorithm for arrays of primitives, providing efficient sorting for large arrays.
Beyond these built-in methods, Java also allows for custom sorting through the Comparator
interface, enabling developers to define their own rules for how elements should be ordered. This is especially useful when dealing with lists of custom objects.
Understanding the underlying principles of these sorting methods and algorithms is essential for choosing the right tool for the job and for writing efficient, effective Java code.
Practical Uses of Java List Sorting
Sorting is not just an academic exercise. It’s a critical operation in many real-world applications. From organizing data in databases, to ordering search results on a website, to scheduling tasks in an operating system, sorting algorithms play a crucial role.
For instance, imagine you’re developing an e-commerce application. You have a list of products that you want to display to the user. Using Java’s sorting methods, you can easily sort this list in various ways – by price, by rating, by the number of reviews, and so on. This not only helps in improving the user experience but also plays a crucial role in the application’s functionality.
Real-World Considerations When Sorting Lists in Java
When sorting lists in Java, especially in larger projects, it’s important to consider the impact on performance. Sorting can be a resource-intensive operation, especially for large lists or complex objects. In such cases, it might be beneficial to use more efficient sorting algorithms or techniques, like parallel sorting.
Further Resources for Mastering Java List Sorting
To delve deeper into the topic of sorting lists in Java, consider exploring these resources:
- IOFlood’s Java List Types Article – Learn about List’s implementations, such as ArrayList and LinkedList.
Exploring List Methods in Java – Learn about List interface methods like size(), contains(), and indexOf().
String Lists in Java – Master handling lists of strings to efficiently process text-based data in Java applications.
Oracle’s Java Tutorials on Collections is a comprehensive guide on Java’s Collections Framework, including sorting.
Baeldung’s Java Sort Methods Article covers various ways to sort collections in Java.
JavaDevJournal’s How to Sort a List in Java Guide covers various approaches to sorting lists in Java.
Recap: Java List Sort Methods
In this comprehensive guide, we’ve taken a deep dive into the world of sorting lists in Java. From understanding the basic Collections.sort()
method to implementing custom sorting with the Comparator
interface, we’ve covered the breadth and depth of this essential Java operation.
We began with the basics, showing how to sort lists of objects that implement the Comparable
interface. We then explored more advanced techniques, such as sorting lists of custom objects using a Comparator
. Along the way, we’ve also discussed alternative methods like Arrays.sort()
, Stream.sorted()
, and third-party libraries like Guava.
We’ve also tackled common challenges and considerations when sorting lists in Java, such as handling null values and performance considerations, providing you with solutions and best practices for each issue.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Collections.sort() | Simple, quick | Can’t handle null values, sorts in ascending order by default |
Comparator | Allows custom sorting rules | More complex, doesn’t handle null values by default |
Arrays.sort() | Good for arrays | Doesn’t handle null values, sorts in ascending order by default |
Stream.sorted() | Functional programming approach | Doesn’t handle null values, requires conversion to and from streams |
Guava’s Ordering | Powerful, fluent API | Requires third-party library |
Whether you’re just starting out with Java or you’re looking to level up your list sorting skills, we hope this guide has given you a deeper understanding of sorting lists in Java.
Sorting is a fundamental operation in many applications, and mastering it can greatly improve the efficiency and effectiveness of your code. Now, you’re well equipped to handle any list sorting task that comes your way in Java. Happy coding!