Python Pandas: How To Read CSV Files
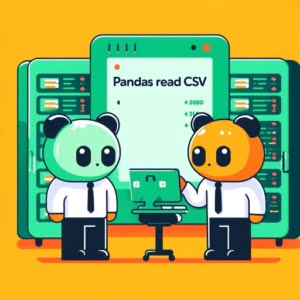
Efficient data handling starts with reading data files seamlessly on our servers at IOFLOOD. The pandas read csv function is a cornerstone in this process, enabling users to read CSV files with ease in Python. Today, we have compiled our insights and best practices on reading CSV files with Pandas, tailored for our programming customers utilizng their dedicated cloud server hosts.
By the end of this guide, you’ll be able to manipulate CSV files with ease, and you’ll have a solid understanding of how pandas can streamline your data analysis workflow.
So let’s dive in and start exploring the power of pandas.
TL;DR: How Do I Read a CSV File with Pandas in Python?
Reading a CSV file with pandas in Python is a straightforward process. You can use the
read_csv()
function in pandas with the syntax,dataframe = pd.read_csv('file.csv')
. Here’s a simple example:
import pandas as pd
df = pd.read_csv('file.csv')
print(df)
# Output:
# (Expected output will be the content of the CSV file, displayed as a pandas DataFrame)
In the above example, we first import the pandas library. We then use the read_csv()
function to read the CSV file and convert it into a DataFrame. The print(df)
command displays the DataFrame in your console.
This basic example illustrates the simplicity of reading CSV files with pandas. However, pandas offers a lot more flexibility and control over how you read your CSV files. So, continue reading for more detailed information and advanced usage scenarios. We’ll cover different parameters you can use with read_csv()
, how to handle common issues, and even explore alternative approaches.
Table of Contents
How to Use: The read_csv() Function
The read_csv()
function in pandas is a versatile tool that allows you to read CSV files in Python. It’s designed to handle a wide range of use cases, from simple CSV files with a few columns to complex files with thousands of rows and columns.
Let’s take a look at a basic example:
import pandas as pd
df = pd.read_csv('file.csv')
print(df)
# Output:
# (Expected output will be the content of the CSV file, displayed as a pandas DataFrame)
In this example, the read_csv()
function reads the CSV file named ‘file.csv’ and converts it into a DataFrame. The DataFrame is a two-dimensional data structure, like a table, that can store data of different types (like integers, strings, and floating-point numbers) in columns. It’s one of the primary data structures in pandas and is extremely versatile.
One of the key advantages of using the read_csv()
function is its simplicity. With just a single line of code, you can read a CSV file and convert it into a format that’s easy to manipulate and analyze. This saves you time and effort, especially when you’re dealing with large datasets.
However, there are a few potential pitfalls you need to be aware of. For instance, the read_csv()
function might not work correctly if your CSV file contains missing values or if the data types of your columns are not consistent. But don’t worry, we’ll cover how to handle these issues in the ‘Troubleshooting and Considerations’ section.
Advanced Usage: Pandas read_csv()
Pandas’ read_csv()
function comes with a host of parameters that can be used to customize how your CSV file is read. Here, we’ll discuss some of the most commonly used parameters: index_col
, header
, and usecols
.
Using index_col
Parameter
The index_col
parameter allows you to specify a column in the CSV file to use as the row labels of the DataFrame. Let’s see an example:
import pandas as pd
df = pd.read_csv('file.csv', index_col='Column1')
print(df)
# Output:
# (Expected output will show 'Column1' as the index of the DataFrame)
In this example, ‘Column1’ from the CSV file is used as the index of the DataFrame. This can be particularly useful when you want to quickly access rows based on the index.
Utilizing header
Parameter
The header
parameter is used to specify the row(s) to use as the column names of the DataFrame. Here’s how you can use it:
import pandas as pd
df = pd.read_csv('file.csv', header=0)
print(df)
# Output:
# (Expected output will show the first row of the CSV file as the column names of the DataFrame)
In this case, the first row (0th index) of the CSV file is used as the column names of the DataFrame.
The Power of usecols
Parameter
The usecols
parameter allows you to specify a subset of columns to be read into the DataFrame. This can be useful when you’re dealing with large CSV files and only need a few specific columns. Here’s an example:
import pandas as pd
df = pd.read_csv('file.csv', usecols=['Column1', 'Column2'])
print(df)
# Output:
# (Expected output will only display 'Column1' and 'Column2' in the DataFrame)
In this scenario, only ‘Column1’ and ‘Column2’ from the CSV file are read into the DataFrame.
These are just a few of the many parameters that read_csv()
offers. Understanding these parameters is key to leveraging the full power of pandas for reading CSV files. Remember, the right use of these parameters can greatly simplify your data analysis workflow.
Alternative Methods to Read CSV Files
While pandas is an incredibly powerful tool for reading CSV files, it’s not the only option. Let’s explore a couple of alternative methods: the csv
module and the numpy
library.
Reading CSV Files with csv
Module
Python’s built-in csv
module provides functionalities to read and write CSV files. Let’s look at an example of how to use it:
import csv
with open('file.csv', 'r') as file:
reader = csv.reader(file)
for row in reader:
print(row)
# Output:
# (Expected output will be each row of the CSV file printed as a list)
In this example, the csv.reader()
function reads the CSV file and returns a reader object which we iterate over to print each row. While this method gives you more control over the reading process, it can be more complex and time-consuming than using pandas, especially with large datasets.
Leveraging numpy
for Reading CSV Files
numpy
is another powerful library that can be used to read CSV files, particularly when your data is numerical. Here’s an example:
import numpy as np
data = np.genfromtxt('file.csv', delimiter=',')
print(data)
# Output:
# (Expected output will be the content of the CSV file displayed as a numpy array)
In this case, the genfromtxt()
function reads the CSV file and returns a numpy array. This method can be faster than pandas for large datasets with numerical data. However, it lacks some of the flexibility and ease of use that pandas offers, particularly when dealing with non-numerical data or missing values.
In conclusion, while pandas’ read_csv()
function is a powerful and flexible tool for reading CSV files, the csv
module and numpy
library offer viable alternatives depending on your specific needs. Understanding these different methods allows you to choose the most effective tool for your data analysis tasks.
Troubleshooting Common Issues
Handling Errors: Pandas CSV Reading
When using pandas to read CSV files, you may encounter some common issues. Here, we’ll discuss how to handle missing values, incorrect data types, and other potential pitfalls.
Handling Missing Values
Missing data can be a common issue when reading CSV files. By default, pandas recognizes certain values like ‘NA’ or ‘NULL’ as missing data. However, you can customize this with the na_values
parameter. Let’s take a look:
import pandas as pd
df = pd.read_csv('file.csv', na_values=['NA', 'NULL', 'Missing'])
print(df)
# Output:
# (Expected output will show 'NA', 'NULL', and 'Missing' as NaN in the DataFrame)
In this example, pandas will recognize ‘NA’, ‘NULL’, and ‘Missing’ as missing values and represent them as NaN in the DataFrame.
Dealing with Incorrect Data Types
Sometimes, pandas might not correctly infer the data types of your columns. You can explicitly specify the data types using the dtype
parameter. Here’s an example:
import pandas as pd
df = pd.read_csv('file.csv', dtype={'Column1': int, 'Column2': float})
print(df)
# Output:
# (Expected output will show 'Column1' as int and 'Column2' as float in the DataFrame)
In this case, ‘Column1’ is read as integers and ‘Column2’ as floating-point numbers.
Remember, understanding these issues and their solutions can help you effectively read and analyze your CSV files with pandas. The more you know about these potential pitfalls, the better you can leverage the power of pandas.
Understanding Pandas and DataFrame
To fully grasp the process of reading CSV files with pandas, it’s essential to understand the basics of the pandas library and the DataFrame object.
The Pandas Library: A Python Powerhouse
Pandas is a software library for Python that provides tools for data manipulation and analysis. It’s built on top of two core Python libraries – Matplotlib for data visualization and NumPy for mathematical operations. Pandas introduces two new data structures to Python – Series and DataFrame, both of which are built on top of NumPy.
Pandas is particularly suited for different kinds of data, such as:
- Tabular data with heterogeneously-typed columns
- Ordered and unordered time series data
- Arbitrary matrix data with row & column labels
- Any other form of observational or statistical data sets
The DataFrame: Your Data’s New Home
A DataFrame is a two-dimensional labeled data structure with columns potentially of different types. You can think of it like a spreadsheet or SQL table, or a dictionary of Series objects. It is generally the most commonly used pandas object.
In the context of our primary keyword ‘pandas read csv’, a DataFrame is the structure that our CSV file will be read into. This makes manipulation and analysis convenient and efficient.
Here’s an example of creating a DataFrame:
import pandas as pd
data = {
'apples': [3, 2, 0, 1],
'oranges': [0, 3, 7, 2]
}
purchases = pd.DataFrame(data)
print(purchases)
# Output:
# apples oranges
# 0 3 0
# 1 2 3
# 2 0 7
# 3 1 2
In this example, we create a DataFrame from a dictionary of lists. Each key-value pair corresponds to a column in the DataFrame. The index of this DataFrame was given to us on creation as the numbers 0-3.
Understanding pandas and DataFrame objects is key to effectively using the ‘pandas read csv’ function. With this foundation, you’re well-equipped to handle CSV files in Python using pandas.
Real-World Usage | Pandas and CSVs
Reading CSV files is a fundamental skill in the field of data analysis and machine learning. CSV files often serve as the starting point for many data analysis projects, containing raw data that needs to be cleaned, analyzed, and visualized.
For instance, in machine learning applications, CSV files often contain the training data for predictive models. Being able to read and manipulate this data with pandas is a critical step in the machine learning workflow.
Exploring Related Concepts
Once you’ve mastered reading CSV files with pandas, there are many related concepts and skills to explore. Data cleaning, for example, is a crucial next step. This involves handling missing values, removing duplicates, and converting data types, among other tasks. Pandas offers a variety of functions for these tasks, such as dropna()
, drop_duplicates()
, and astype()
.
Data visualization is another key skill in data analysis. Libraries like Matplotlib and Seaborn work seamlessly with pandas DataFrames, allowing you to create a wide range of visualizations to better understand your data.
Further Resources for Pandas Library
To deepen your understanding of pandas and data analysis in Python, consider exploring resources like the Pandas Documentation, Python Data Science Handbook, and online courses on platforms like Coursera and edX. These resources offer in-depth tutorials, examples, and exercises that can help you become a proficient data analyst or data scientist.
Mastering ‘pandas read csv’ is just the beginning of your data analysis journey. There’s a whole world of data out there waiting for you to explore.
You can also find more helpful resources the Pandas library here on our blog:
- Practical Pandas: Implementing Data Analysis in Real-world Projects: Learn about the practical applications of Pandas in various industries and projects through this informative resource.
Converting a Pandas DataFrame to a List: This article explains different methods to convert a Pandas DataFrame to a list in Python, providing examples for easy understanding.
Using the Pandas concat() Function for Data Concatenation: This guide dives into the details of using the concat() function in Pandas to concatenate data from multiple DataFrames.
pandas.read_csv() – pandas API Reference: Official documentation for the read_csv() function in Pandas, providing details on how to read a CSV file into a DataFrame.
Python – Read CSV using Pandas read_csv(): A GeeksforGeeks article that explains how to use the read_csv() function in Pandas to read CSV files in Python.
Pandas CSV File Reading and Writing: A Comprehensive Guide: A comprehensive guide on w3schools.com that covers various ways to read and write CSV files using Pandas in Python.
Recap: Read CSV Files with Pandas
Throughout this guide, we’ve explored the process of reading CSV files using pandas in Python. We’ve seen how the read_csv()
function can simplify this task, turning a CSV file into a pandas DataFrame with just a single line of code.
import pandas as pd
df = pd.read_csv('file.csv')
print(df)
# Output:
# (Expected output will be the content of the CSV file, displayed as a pandas DataFrame)
We delved into the different parameters of the read_csv()
function, such as index_col
, header
, and usecols
, and how they can provide more control over how your CSV file is read.
We also discussed common issues you might encounter when reading CSV files with pandas, such as handling missing values and dealing with incorrect data types. We provided solutions and workarounds for these issues, ensuring a smooth data analysis process.
Beyond pandas, we explored alternative methods to read CSV files in Python, such as the csv
module and the numpy
library. These alternatives offer different advantages and can be more suitable depending on your specific needs.
In essence, understanding how to read CSV files with pandas is a fundamental skill in data analysis and machine learning. It’s the first step in turning raw data into valuable insights. So keep exploring, keep learning, and keep unlocking the power of your data with pandas.