Python Language for Beginners: Comprehensive Guide
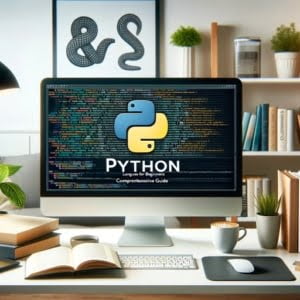
Welcome to the world of Python programming, a high-level, general-purpose language that’s making significant strides in the tech industry. Python is renowned for its user-friendly syntax and broad applications, making it a preferred choice for both novices and seasoned professionals.
Python’s inception was driven by the need for a more accessible and readable language. Over time, it has evolved into a fundamental tool in diverse technology sectors, from web development to data analysis, showcasing its impressive versatility.
But why should you, a beginner, consider learning Python? The reason is clear: Python serves as an excellent entry point into programming. Its simplicity doesn’t compromise its power, making it an ideal starting point for beginners aiming to acquire robust programming skills. So, fasten your seatbelts as we embark on an enlightening journey into the world of Python programming.
TL;DR: Why should a beginner consider learning Python?
Python is an excellent gateway into programming due to its simplicity and power. It’s user-friendly, versatile, and widely used in various tech sectors, making it a valuable skill for beginners. For a deeper dive into Python’s capabilities and how to leverage them, continue reading this comprehensive guide.
Table of Contents
Python: An Introduction
Python, a high-level, general-purpose programming language, was brought to life by Guido van Rossum and first saw the light of day in 1991. The primary objective behind Python’s inception was to conceive a language that prioritizes code readability and empowers programmers to express ideas in fewer lines of code, compared to languages like C++ or Java.
Example of Python’s simplicity compared to C++ or Java:
Python:
# Python
print('Hello, World!')
Java:
// Java
class HelloWorld {
public static void main(String[] args) {
System.out.println('Hello, World!');
}
}
Python: Key Syntax and Features
Python’s simplicity and versatility make it an excellent starting point for anyone looking to delve into programming. However, before you write your first line of code, it’s crucial to familiarize yourself with the key syntax and features of Python.
These range from fundamental elements like data structures and loops to more advanced concepts such as functions and libraries. In this section, we take you through some essential Python elements you should master to kickstart your programming journey.
Python’s Syntax
Python’s syntax is clean and readable, making it an easy language to pick up, especially for beginners. Syntax, in programming terms, refers to the set of rules that dictate how programs in a specific language must be written. Understanding syntax is important as it ensures that your code is valid and functional.
See our Python Syntax Cheat Sheet for a breakdown of common syntax elements and a well organized example program that highlights the various syntax options.
Here are some of the crucial syntax concepts to familiarize yourself with when learning Python:
Concept | Description |
---|---|
Indentation | Python uses whitespace (indentation) to denote blocks of code. Unlike many other languages that use brackets or keywords for this. |
Variables | Variables are containers for storing data. Python has no command for declaring a variable; it’s created when you first assign a value to it. |
Comments | Start comments with a ‘#’ in Python. Python will ignore the rest of the line. It’s commonly used to make notes or explain sections of your code. |
Statements | Instructions that a Python interpreter can execute are called statements. An example might be a = 1 + 2 . |
Data Types and Structures
Python boasts a diverse range of built-in data types and structures which allows you to store and manipulate data in different ways.
- Data types categorize and specify the type of values a variable can hold, like integer, string, boolean, etc.
Data structures, on the other hand, enable you to organize, store, and manage data efficiently.
Here’s a brief introduction to some necessary Python data types and structures:
Type/Structure | Description | Mutable/Immutable |
---|---|---|
Integers | Represented as int in Python, it stores numeric data. | Immutable |
Strings | Python’s str data type is used to store strings which are sequences of character data. | Immutable |
Booleans | Boolean values are either True or False , used often in conditional statements. | Immutable |
Lists | A data structure which holds an ordered collection of items, which can be of any type. | Mutable |
Tuples | Similar to lists, but unlike lists are immutable (cannot be changed). | Immutable |
Dictionaries | An unordered collection of data in a key:value pair form. | Mutable |
Mutable vs Immutable Data Types
You can get into trouble if you don’t understand the function and consequences of mutable data types in Python. With mutable data types, variables you pass to a function can be edited within that function, and those edits persist across the rest of the program. This is probably the biggest source of mistakes and bugs for beginner Python developers, so it is essential to learn the differences between mutable vs immutable data types.
Control Structures
Control structures guide the flow of a Python program, allowing you to execute or skip blocks of code based on certain conditions or loops. Mastering control structures allows you to incorporate logic into your code.
Here’s a brief look at some fundamental Python control structures:
Structure | Description |
---|---|
If Statement | An if statement is used to test a condition and perform a task based on its outcome. |
For Loop | For loop iterates over a sequence (like a list or string), executing a block of code until the sequence is exhausted. |
While Loop | A while loop in Python repeatedly executes a target statement as long as a given condition remains true. |
Break and Continue | Break is used to exit a for loop or a while loop, whereas continue is used to skip the current block, and return to the “for” or “while” statement. |
Functions
Functions in Python are blocks of reusable code that perform a specific task. Functions make your code more efficient and easier to manage, test, and debug. Python has built-in functions like print()
, len()
, etc., but you can also define your own functions using the def
keyword.
Here’s a brief overview of functions in Python:
Concept | Description |
---|---|
Defining Functions | To define a function in Python, the def keyword is used. It’s then followed by a function name and parentheses which may include parameters. |
Calling Functions | To execute a function, you need to call it. This can be done by using the function name followed by parentheses. |
Return Statement | Return is used to exit a function and return a value. |
See our article on python functions for a more comprehensive rundown of built in python functions as well as commonly used libraries.
Libraries
A significant part of Python’s appeal is its broad, robust standard library. Python’s standard library is packed with a wide range of modules that provide tools for a variety of tasks, from parsing XML, CSV, and yaml files to connecting to web servers.
Alongside these are countless third-party libraries that further expand Python’s capabilities, such as advanced mathematical computations with libraries like matplotlib, numpy, scipy, and pandas.
Here are a few essential Python libraries:
Library | Description |
---|---|
NumPy | Provides support for large, multi-dimensional arrays and matrices, and a collection of mathematical functions to operate on these arrays. |
Pandas | An open-source library providing high-performance, easy-to-use data structures and data analysis tools. |
Matplotlib | A plotting library for the Python programming language and its numerical mathematics extension NumPy. |
Django | A high-level web framework that encourages rapid development and clean, pragmatic design. |
Requests | An elegant and simple HTTP library for Python, built for human beings. |
FastAPI | A modern, fast web framework for building APIs with Python 3.7+ based on standard Python type hints. |
PySpark | An interface for Apache Spark in Python. It not only allows you to write Spark applications using Python APIs, but also provides the PySpark shell for interactively analyzing your data in a distributed environment. |
Beautiful Soup | A library for pulling data out of HTML and XML files. It provides idiomatic ways of navigating, searching, and modifying the parse tree. |
Jinja | A modern and designer-friendly templating engine for Python programming language. It is used to create HTML, XML or other markup formats that are returned to the user via an HTTP request. |
Openpyxl | A Python library to read/write Excel 2010 xlsx/xlsm/xltx/xltm files. It was born from lack of an existing library to read/write natively from Python the Office Open XML format. |
Polars | An in-memory DataFrame library implemented in Rust and Python. It is designed to perform data manipulation operations at speed and scale. |
Python Regex | A module in Python that provides support for regular expressions. It allows for searching, matching, and manipulating strings based on specific patterns. |
Flask | A lightweight WSGI web application framework in Python. It is designed to make getting started quick and easy, with the ability to scale up to complex applications. |
Error Handling
Error handling is a crucial aspect of programming in any language, including Python. Python has several built-in exceptions that are raised when your program encounters an error. Understanding how to handle these errors can save you a lot of trouble debugging your code.
Some basic concepts related to error handling in Python include:
Concept | Description |
---|---|
Exceptions | Exceptions are raised when an error occurs. Python includes several built-in exceptions, such as KeyError occurs when a dictionary is accessed with a key that does not exist. |
Try/Except | A try block is used to enclose the code that might throw an exception. The except block is used to catch and handle the exception(s) that are encountered in the try block. |
Finally | The finally keyword in Python is used to specify a block of code to be executed after a try block, irrespective of whether an error was encountered or not. |
File Operations
Operating with files is a common task in Python programming. Whether it’s reading data from a CSV file or writing logs to a text file, understanding file operations is vital.
Here are some fundamental file operation concepts in Python:
Concept | Description |
---|---|
Open Function | The open() function is used to open a file. It takes two parameters: file name and mode. |
Read and Write Operations | The read() method reads the whole file, and write() method writes any string to an opened file. |
Close Method | The close() method closes an opened file. It’s a good practice to always close the file when you’re done with it. |
Pathlib | The pathlib module offers classes representing filesystem paths with semantics appropriate for different operating systems. |
ZipFile | The zipfile module provides tools to create, read, write, append, and list a ZIP file. |
Create File | Various ways to create a file in Python, including using the open() function with write mode and pathlib’s touch() method. |
Check if File Exists | Techniques for checking whether a file or directory exists using methods like os.path.exists() and pathlib.Path().exists() . |
Copy File | Provides different methods of copying a file in Python, such as using shutil , os , and more. |
Delete File | Various methods to delete or remove a file or folder in Python using modules like os and shutil . |
List Files in Directory | Techniques to list files in a directory using methods from modules like os and glob . |
Read File Line by Line | Discusses methods for reading a file line by line in Python, which is memory efficient. |
Rename File | Showcases methods for renaming files in Python using os.rename() , shutil.move() , and other approaches. |
These topics provide a comprehensive overview for someone just starting out with Python, and forms a solid foundation to delve into more complex topics in the field.
Python’s Object-Oriented Programming
While Python is versatile and can support different programming styles, it’s fundamentally an object-oriented language. Understanding object-oriented programming (OOP) concepts can greatly improve your effectiveness in writing Pythonic code.
Here are key OOP concepts in Python:
Concept | Description |
---|---|
Classes and Objects | Classes are the blueprints for creating Objects (an instance of a class). |
Inheritance | It’s the capability of one class to derive or inherit properties from another class. |
Encapsulation | Encapsulation describes the idea of wrapping data and the methods that work on data within one unit. |
Modules and Packages
Modules and packages are foundational to managing and organizing large code bases. A Python module is a .py file containing executable code, while packages are ways of organizing related Python modules into a single directory hierarchy.
Concept | Description |
---|---|
Creating Modules | You can create your own module by creating a new .py file and importing it using the filename. |
Importing Modules | Modules can be imported into other modules or into the main Python script using the import keyword. |
Python Packages | A package is a way of organizing related modules into a single directory hierarchy. It helps to organize and encapsulate functionality, which makes maintaining larger code bases much easier. |
PIP | PIP is the most popular Python Package Manager, used for installing and updating packages. |
Poetry | Poetry is a tool for dependency management and packaging in Python. It allows you to declare the libraries your project depends on and it will manage (install/update) them for you. |
Python Version Manager | There are many tools and approaches for managing multiple versions of Python, such as pyenv, Pythonbrew, and Anaconda. |
Pipenv | Pipenv is a packaging tool for Python that automatically creates and manages a virtual environment for your projects. |
Python’s Nature Unveiled
Python is an interpreted language, meaning its code is executed line by line, making debugging more straightforward and the language more adaptable. Python also boasts an interactive nature. You can interact directly with the Python interpreter, a feature that facilitates easy testing and debugging of code snippets.
Python is designed on the principles of object-oriented programming, supporting multiple inheritance and classes. This feature helps create complex data structures and reusable code, leading to more efficient and manageable programs.
Dynamic Typing and Garbage Collection
Python is dynamically-typed, meaning the type of a variable is checked during runtime. This provides flexibility as there’s no need to declare the variable type during its creation.
Python also features garbage collection, which automatically manages and recycles memory no longer in use. This functionality helps prevent memory leaks, making Python more beginner-friendly.
Example of Python’s dynamic typing and garbage collection:
# Dynamic Typing
x = 5
print(type(x))
x = 'Hello'
print(type(x))
# Garbage Collection
import gc
print('Unreachable objects:', gc.collect())
print('Remaining Garbage:', gc.garbage)
Python’s Philosophy: Emphasis on Code Readability
Python’s design philosophy is elegantly encapsulated in the Zen of Python, a set of 19 guiding principles. ‘Readability counts’ is a key principle, explaining why Python’s syntax is clean and easy to comprehend. This focus on code readability makes Python a preferred language among beginners and experts alike, enabling efficient coding and easier maintenance and updating of code.
Python’s Rising Demand in the Job Market
Python has rapidly emerged as one of the most in-demand programming languages in the job market. As reported by the TIOBE Index, Python ranks among the top 3 programming languages in 2023. Its extensive use across diverse fields such as web development, data analysis, artificial intelligence, and more has fueled a surge in the demand for Python programmers.
Rank | Programming Language |
---|---|
1 | Python |
2 | C |
3 | Java |
Job Opportunities for Python Programmers
Python’s versatility paves the way for a myriad of job roles. As a Python programmer, your potential roles could range from a software engineer to a data analyst, data scientist, or even a machine learning engineer.
Python’s common use in network programming, game development, and scientific computing further expands the career possibilities, making Python an excellent choice for those aspiring to enter the tech industry.
Pay for Python Programmers
Python programmers are rewarded well for their skills. As per PayScale, the average salary for a Python developer in the United States hovers around $81,098 in 2023.
However, this figure can fluctuate based on factors such as experience, location, and the specific field of work. For instance, Python developers engaged in data science or machine learning generally earn more than their counterparts in web development.
Field | Average Salary (USD) |
---|---|
Data Science Manager | 145,000 |
Machine Learning | 112,000 |
Web Development | 74,000 |
Python’s Influence in Emerging Technologies
Python’s influence in the tech industry transcends traditional programming. It finds widespread application in burgeoning fields like artificial intelligence (AI) and machine learning (ML).
Python’s extensive library support, including libraries like TensorFlow and Keras, makes it a fitting language for these domains. Moreover, Python’s simplicity and readability render complex AI and ML algorithms more manageable and easier to implement.
Python: A Crucial Skill in Tech
Python’s versatility renders it a crucial skill across various tech domains. Whether your interest lies in web development, data science, AI, or any other tech field, Python is a skill that will undoubtedly benefit you. Its extensive use and growing demand in the job market make it a wise choice for anyone aiming to enhance their tech career.
The Popularity and Ease of Learning Python
Python has been on a steady growth trajectory in popularity for several years now. As per the PYPL Popularity of Programming Language Index, Python holds the crown as the most popular programming language as of 2023.
But what fuels Python’s popularity? A key factor is its ease of learning. Python’s syntax, designed for readability and simplicity, makes it an ideal language for beginners. It’s no surprise that many introductory programming courses opt for Python as their language of choice.
Python’s Wide-Ranging Applications
Python’s versatility is showcased in its broad spectrum of applications. It’s extensively used in web and internet development, facilitating the creation of frameworks like Django and Pyramid, and micro-frameworks such as Flask and Bottle. Python’s standard library supports various internet protocols like HTML and XML, JSON, email processing, and more.
In the realm of scientific and numeric computing, Python is employed in SciPy for mathematics, science, and engineering applications, and in Pandas for data analysis and modeling. The versatility of Python also extends to educational platforms, desktop GUIs, and software development.
Python’s Support for GUI and Database Interfaces
Python supports GUI applications, offering a variety of toolkits, including Tk, wxWidgets, and others. It also complies with the API 2.0 standards for database interfaces, supporting a wide range of database servers.
Example of Python’s support for GUI and database interfaces:
# GUI Application
from tkinter import Tk, Label
root = Tk()
Label(root, text='Hello, World!').pack()
root.mainloop()
# Database Interface
import sqlite3
conn = sqlite3.connect('example.db')
Python in Data Analysis and Visualization
Python is also extensively used in data analysis and visualization. Libraries like Pandas, Numpy and Matplotlib are commonly used in the data science field. They are wide-ranging tools capable of data cleaning, analysis, and powerful visualization capabilities. Seaborn, Plotly and Bokeh are some other libraries offering flexible interfaces for producing superior statistical graphics.
Example of Python in data analysis and visualization:
# Data Analysis with pandas and numpy
import pandas as pd
import numpy as np
df = pd.DataFrame(np.random.rand(10, 5), columns=['A', 'B', 'C', 'D', 'E'])
df.describe()
# Data Visualization with Matplotlib
import matplotlib.pyplot as plt
plt.plot(df['A'], df['B'])
plt.show()
Python in Machine Learning and Artificial Intelligence
Python’s simplicity and readability have made it a popular choice for artificial intelligence and machine learning. Libraries like Scikit-Learn, TensorFlow and PyTorch offer a wide range of algorithms for building and training machine learning systems. These libraries support an array of algorithms for regression, classification, clustering, dimension reduction, and more.
Example of Python in machine learning:
# Machine Learning Application with sklearn
from sklearn import svm
from sklearn import datasets
clf = svm.SVC()
X, y = datasets.load_iris(return_X_y=True)
clf.fit(X, y)
Python in Web Scraping
Python is widely used for web scraping because of libraries like Beautiful Soup and Scrapy. These libraries are perfect tools for collecting the data you need from online resources.
Beautiful Soup is used for parsing HTML and XML documents, and it works well for web scraping. Scrapy, on the other hand, is an open-source and collaborative web scraping framework for Python.
Example of Python in web scraping:
# Web Scaping with Beautiful Soup
from bs4 import BeautifulSoup
import requests
URL = "http://www.example.com"
r = requests.get(URL)
soup = BeautifulSoup(r.content, 'html5lib') # If this line causes an error, run 'pip install html5lib' or install html5lib
print(soup.prettify())
Python in Network Programming
Python provides low-level networking to high-level networking e.g. Python’s standard library provides select which directly interfaces with operating system’s I/O mechanisms for efficient networking. Twisted, a framework for asynchronous network programming and Tornado, a lightweight version of Django optimized for speed and performance, are also used in network programming.
Example of Python in network programming:
# Network programming with socket
import socket
s = socket.socket()
host = socket.gethostname()
port = 12345
s.bind((host, port))
s.listen(5)
while True:
c, addr = s.accept()
print('Got connection from', addr)
c.send('Thank you for connecting')
c.close()
Concluding Thoughts: The Python Programming Journey
In this detailed guide, we embarked on an enlightening journey into the world of Python programming, a high-level, general-purpose language that’s making a substantial mark in the tech industry. We delved into Python’s intriguing history, its unique characteristics, and its diverse applications, highlighting why it’s a preferred choice among beginners and seasoned professionals alike.
We considered the key syntax, features, and functions of Python, linking to many resources to learn the fundamentals of programming in python.
We examined the promising job market for Python programmers, discussing the increasing demand and the array of potential job roles you could explore. Python’s versatility renders it a valuable skill across diverse tech fields, from web development to data science and beyond.
We highlighted why Python stands out as an excellent language to learn, emphasizing its soaring popularity, ease of learning, and robust libraries. Python’s interactive mode and comprehensive standard library make it a ‘batteries included’ language, ideal for rapid application development.
We explored Python’s key characteristics, including its interpreted nature, dynamic typing, object-oriented design, and automatic memory management. We also discussed its wide-ranging applications, its support for GUI programming and database interfaces, and its scalability and extendability.
Python’s simplicity, coupled with its power and versatility, make it a go-to language for a multitude of applications. Whether you’re a novice aiming to break into programming or an experienced developer looking to broaden your skills, Python is a stellar choice. So, why wait? Dive in and kickstart your Python journey today!